How to set the background color of chip in kotlin android , You can change the color of chip background
Example of chip background color in kotlin
MainActivity.kt
package com.jigopost.chipbackground
import android.content.res.ColorStateList
import android.graphics.Color
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.core.view.children
import com.google.android.material.chip.Chip
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get chip group initially checked chips
handleSelection()
// set checked change listener for each chip on chip group
chipGroup.children.forEach {
val chip = it as Chip
chip.setOnCheckedChangeListener { buttonView, isChecked ->
handleSelection()
}
// set chip background color programmatically
// both for chip checked and unchecked states
chip.chipBackgroundColor = setChipBackgroundColor(
checkedColor = Color.parseColor("#8DB600"),
unCheckedColor = Color.parseColor("#FF9966")
)
}
}
// function to get chip group checked chips
private fun handleSelection(){
textView.text = "Checked Chips : "
chipGroup.checkedChipIds.forEach{
textView.append("\n${findViewById<Chip>(it).text}")
}
}
}
// function to generate color state list for chip background
fun setChipBackgroundColor(
checkedColor: Int = Color.GREEN,
unCheckedColor: Int = Color.RED
): ColorStateList{
val states = arrayOf(
intArrayOf(android.R.attr.state_checked),
intArrayOf(-android.R.attr.state_checked)
)
val colors = intArrayOf(
// chip checked background color
checkedColor,
// chip unchecked background color
unCheckedColor
)
return ColorStateList(states, colors)
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FEFEFA"
tools:context=".MainActivity">
<com.google.android.material.chip.ChipGroup
android:id="@+id/chipGroup"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="8dp"
app:singleSelection="false"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.chip.Chip
android:id="@+id/chipRed"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:text="Red" />
<com.google.android.material.chip.Chip
android:id="@+id/chipGreen"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:text="Green" />
<com.google.android.material.chip.Chip
android:id="@+id/chipYellow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Action"
android:checkable="true"
android:text="Yellow" />
<com.google.android.material.chip.Chip
android:id="@+id/chipBlue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:enabled="false"
android:text="Blue" />
<com.google.android.material.chip.Chip
android:id="@+id/chipBlack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:text="Black" />
<com.google.android.material.chip.Chip
android:id="@+id/chipMagenta"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checkable="true"
android:text="Magenta" />
</com.google.android.material.chip.ChipGroup>
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:fontFamily="sans-serif-condensed-medium"
android:gravity="center"
android:padding="8dp"
android:textColor="#4F42B5"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chipGroup"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
Output
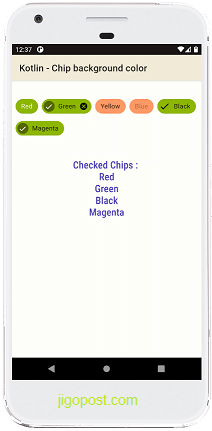