How to Volley image request, How to Volley image request example, kotlin Volley image request, android kotlin Volley image request.
MainActivity.kt
package com.jigopost.volley
import android.graphics.Bitmap
import android.os.Bundle
import android.text.method.ScrollingMovementMethod
import android.view.View
import android.widget.ImageView
import androidx.appcompat.app.AppCompatActivity
import com.android.volley.toolbox.ImageRequest
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// make text view content scrollable
textView.movementMethod = ScrollingMovementMethod()
// url to fetch/download image
val imageUrl = "https://images.pexels.com/photos/1085551/" +
"pexels-photo-1085551.jpeg?" +
"auto=compress&cs=tinysrgb&dpr=2&h=750&w=1260"
// fetch image from url using volley network library
button.setOnClickListener {
progressBar.visibility = View.VISIBLE
// request a image response from the provided url
val imageRequest = ImageRequest(
imageUrl,
{bitmap -> // response listener
textView.text = "Image downloaded successfully!"
imageView.setImageBitmap(bitmap)
progressBar.visibility = View.INVISIBLE
},
0, // max width
0, // max height
ImageView.ScaleType.CENTER_CROP, // image scale type
Bitmap.Config.ARGB_8888, // decode config
{error-> // error listener
textView.text = error.message
progressBar.visibility = View.INVISIBLE
}
)
VolleySingleton.getInstance(applicationContext)
.addToRequestQueue(imageRequest)
}
}
}
VolleySingleton.kt
package com.jigopost.volley
import android.content.Context
import android.graphics.Bitmap
import android.util.LruCache
import com.android.volley.Request
import com.android.volley.RequestQueue
import com.android.volley.toolbox.ImageLoader
import com.android.volley.toolbox.Volley
class VolleySingleton constructor(context: Context) {
companion object {
@Volatile
private var INSTANCE: VolleySingleton? = null
fun getInstance(context: Context) =
INSTANCE ?: synchronized(this) {
INSTANCE ?: VolleySingleton(context).also {
INSTANCE = it
}
}
}
val imageLoader: ImageLoader by lazy {
ImageLoader(requestQueue,
object : ImageLoader.ImageCache {
private val cache = LruCache<String, Bitmap>(20)
override fun getBitmap(url: String): Bitmap {
return cache.get(url)
}
override fun putBitmap(url: String, bitmap: Bitmap) {
cache.put(url, bitmap)
}
})
}
private val requestQueue: RequestQueue by lazy {
// applicationContext is key, it keeps you from leaking the
// Activity or BroadcastReceiver if someone passes one in.
Volley.newRequestQueue(context.applicationContext)
}
fun <T> addToRequestQueue(req: Request<T>) {
requestQueue.add(req)
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:text="Fetch Image"
android:backgroundTint="#3B2F2F"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:visibility="invisible"
app:layout_constraintBottom_toBottomOf="@+id/button"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="@+id/button" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="12dp"
android:fontFamily="sans-serif-condensed-medium"
android:padding="8dp"
android:textColor="#4F42B5"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
tools:text="TextView" />
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="300dp"
android:layout_marginTop="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView"
tools:srcCompat="@tools:sample/avatars" />
</androidx.constraintlayout.widget.ConstraintLayout>
Add to dependency to app.gradle [dependencies]
// volley network library
implementation 'com.android.volley:volley:1.1.1'
Output
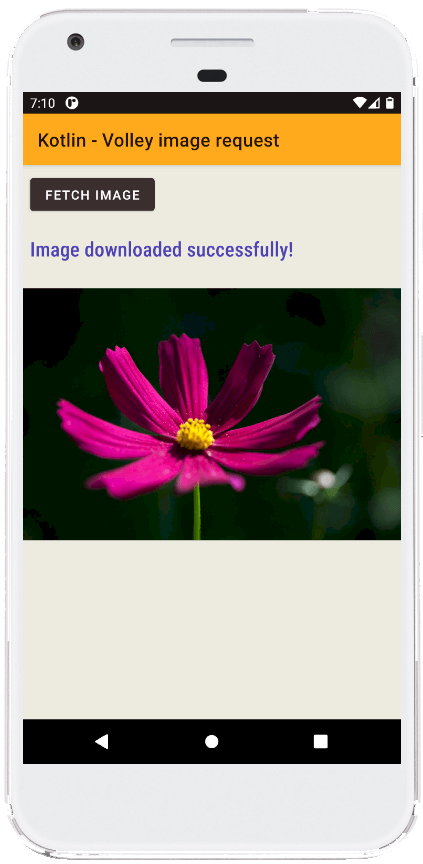