In this tutorial we will learn how to open new activity when a button is clicked using android studio. move From one Activity to another Activity in Android Studio. When button in an activity(Main Activity) is clicked the new activity(New Activity) will be opened..
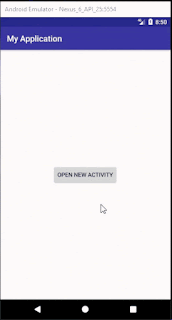
Step 1: Create a new Project of open new project:
How to create a new project?
Step 2: Create a new Activity(e.g. Empty Activity)
Note: after clicking we will move to this activity..
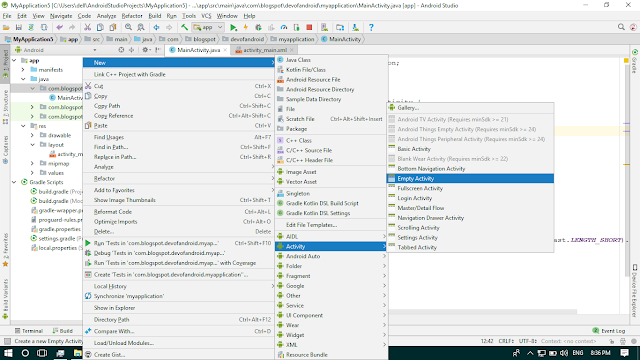
Step 3: activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Open New Activity" />
</LinearLayout>
Step 4: MainActivity.java
package com.jigopost.myapplication;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//initialize
Button mButton = (Button)findViewById(R.id.button);
//handle onClick
mButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//intent to start NewActivity
startActivity(new Intent(MainActivity.this, NewActivity.class));
}
});
}
}
Step 5: actiavity_new.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".NewActivity">
<TextView
android:text="New Activity"
android:textSize="30sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
Step 6: NewActivity.java
package com.jigopost.myapplication;
import android.support.v7.app.ActionBar;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
public class NewActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_new);
}
}
Step 7: Output
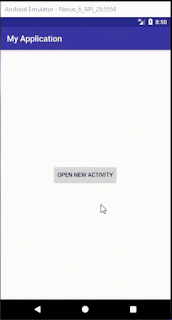