How to programatically resize and show them?. All of these attributes can be set in code on each ImageButton at runtime.
Change size of image button android studio
Now we give you example of Change size of image button android studio with code.
activity_main.xml
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/rl"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
tools:context=".MainActivity"
android:background="#b1b3a9"
>
<!-- ImageButton with default size, no scaling -->
<ImageButton
android:id="@+id/ib"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/image_button2"
/>
<!-- ImageButton scaled, width 100dp and height 50dp by XML -->
<!-- ImageButton scale type fit center by XML -->
<ImageButton
android:id="@+id/ib2"
android:layout_width="100dp"
android:layout_height="50dp"
android:src="@drawable/image_button2"
android:layout_alignParentRight="true"
android:scaleType="fitCenter"
/>
<!-- ImageButton scaled, width 200dp and height 100dp by XML -->
<!-- ImageButton scale type fit x y by XML -->
<ImageButton
android:id="@+id/ib3"
android:layout_width="200dp"
android:layout_height="100dp"
android:src="@drawable/image_button2"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:scaleType="fitCenter"
/>
<!-- ImageButton scaling programmatically in java code -->
<ImageButton
android:id="@+id/ib4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/image_button2"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"
/>
</RelativeLayout>
MainActivity.java
package com.jigopost.resizebutton;
import android.os.Bundle;
import android.app.Activity;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.RelativeLayout;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the widgets reference from XML layout
final RelativeLayout rl = (RelativeLayout) findViewById(R.id.rl);
final ImageButton ib = (ImageButton) findViewById(R.id.ib);
final ImageButton ib2 = (ImageButton) findViewById(R.id.ib2);
final ImageButton ib3 = (ImageButton) findViewById(R.id.ib3);
final ImageButton ib4 = (ImageButton) findViewById(R.id.ib4);
// Get the last ImageButton's layout parameters
RelativeLayout.LayoutParams params = (RelativeLayout.LayoutParams) ib4.getLayoutParams();
// Set the height of this ImageButton
params.height = 200;
// Set the width of that ImageButton
params.width = 350;
// Apply the updated layout parameters to last ImageButton
ib4.setLayoutParams(params);
// Set the ImageButton image scale type for fourth ImageButton
ib4.setScaleType(ImageView.ScaleType.FIT_XY);
}
}
Output
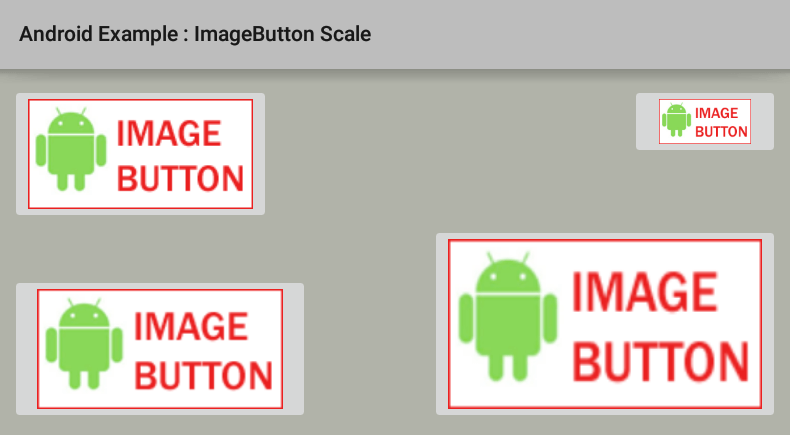