SharedPreferences | Android Studio | Java
SharedPreferences is one of the types of saving data in Android Devices. You can save String, int, boolean, long, float, and Set<String> types of data in SharedPreferences. In SharedPreferences data is saved in the key-value form. If you have a relatively small collection of key-values that you’d like to save, then you should use the SharedPreferences APIs. You can Add, Edit/Modify and Remove data from the SharedPreferences easily.
To get the access to the preferences, we have the three APIs to choose from:
getPreferences() : used from within your Activity, to access the activity-specific preferences.getSharedPreferences() : used from within your Activity (or other application Context), to access the application-level preferences.getDefaultSharedPreferences() : used on the PreferenceManager, to get the shared preferences that work in concert with Android’s overall preference framework.
Step 1: Create a new project OR Open your existing project
Step 2: Code
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp"
tools:context=".MainActivity">
<EditText
android:id="@+id/nameEt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Name"
android:inputType="textPersonName|textCapWords" />
<EditText
android:id="@+id/ageEt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Age"
android:inputType="date" />
<EditText
android:id="@+id/emailEt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Email"
android:inputType="textEmailAddress" />
<EditText
android:id="@+id/passwordEt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Password"
android:inputType="textPassword" />
<CheckBox
android:id="@+id/rememberCb"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Remember" />
<Button
android:id="@+id/saveBtn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Save" />
</LinearLayout>
MainActivity.java
package com.jigopost.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
//declare views
private EditText nameEt, ageEt, emailEt, passwordEt;
private CheckBox rememberCb;
private Button saveBtn;
//variables
String name, email, password;
int age;
boolean isRemembered;
//shared pref
SharedPreferences sharedPreferences;
SharedPreferences.Editor spEditor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//init views
nameEt = findViewById(R.id.nameEt);
ageEt = findViewById(R.id.ageEt);
emailEt = findViewById(R.id.emailEt);
passwordEt = findViewById(R.id.passwordEt);
rememberCb = findViewById(R.id.rememberCb);
saveBtn = findViewById(R.id.saveBtn);
//init share pref
sharedPreferences = getSharedPreferences("USER_INFO_SP", MODE_PRIVATE);
//get data from shared preferences
name = sharedPreferences.getString("NAME", "");
age = sharedPreferences.getInt("AGE", 0);
email = sharedPreferences.getString("EMAIL", "");
password = sharedPreferences.getString("PASSWORD", "");
isRemembered = sharedPreferences.getBoolean("REMEMBER", false);
//set data to views
nameEt.setText(name);
ageEt.setText("" + age);
emailEt.setText(email);
passwordEt.setText(password);
rememberCb.setChecked(isRemembered);
//click to input data from views
saveBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//get data from views
name = "" + nameEt.getText().toString().trim();
age = Integer.parseInt(ageEt.getText().toString().trim());
email = "" + emailEt.getText().toString().trim();
password = "" + passwordEt.getText().toString().trim();
if (rememberCb.isChecked()) {
isRemembered = true;
//save data to shared preferences
spEditor = sharedPreferences.edit();
spEditor.putString("NAME", name);
spEditor.putInt("AGE", age);
spEditor.putString("EMAIL", email);
spEditor.putString("PASSWORD", password);
spEditor.putBoolean("REMEMBER", true);
spEditor.apply();
Toast.makeText(MainActivity.this, "Info is remembered...", Toast.LENGTH_SHORT).show();
} else {
isRemembered = false;
//don't save | remove data from shared preferences
spEditor = sharedPreferences.edit();
spEditor.clear();
spEditor.apply();
Toast.makeText(MainActivity.this, "Info is not remembered...", Toast.LENGTH_SHORT).show();
}
}
});
}
}
Step 3: Run Project
Output
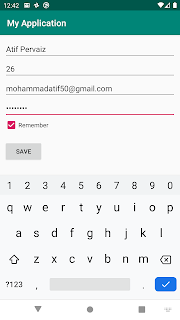
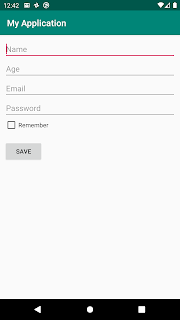